Connect to your API from your frontend
The remaining chapters of this tutorial walk through building our application frontend, which uses Apollo Client to communicate with the GraphQL server we just built:
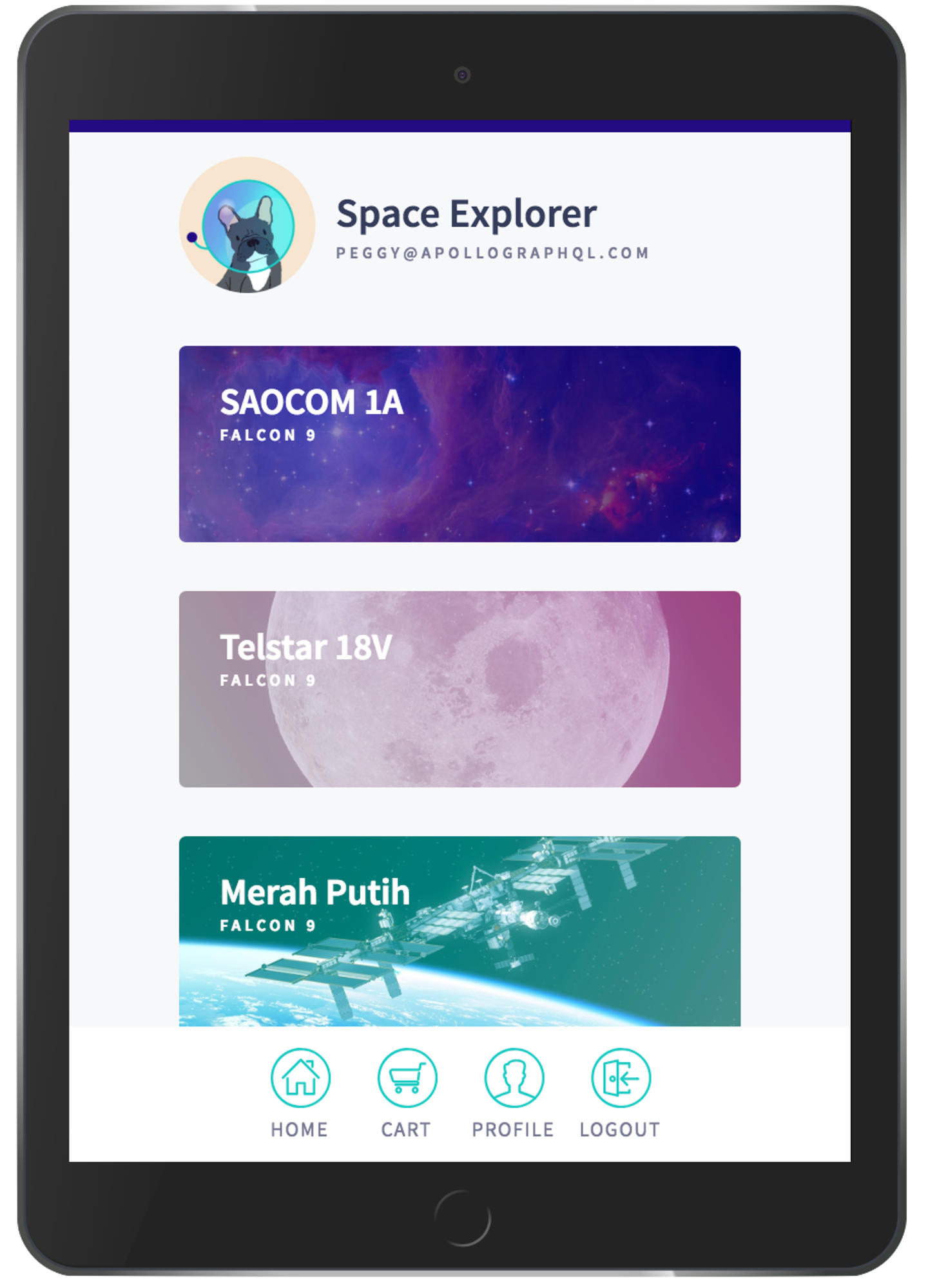
Apollo Client is a comprehensive state management library for Typescript & JavaScript. It enables you to use GraphQL to manage both local and remote data. Apollo Client is view-layer agnostic, so you can use it with any of your favorite view layers such as: React, React Native, Vue, Ember Angular, Svelte, Solid.js, Web Components, or vanilla JS.
This tutorial uses React (which Apollo Client's core library supports out of the box), but the underlying concepts are the same for every view layer.
Setup
We'll be working in the start/client/
directory of our tutorial repo (clone the repo here if you skipped the server portion). From that directory, run:
npm install
Along with installing other dependencies, this installs the @apollo/client
package, which includes all of the Apollo Client features we'll use.
Initialize ApolloClient
Let's create our instance of ApolloClient
in the start/client
directory by pasting the following into src/index.tsx
import { ApolloClient, gql } from "@apollo/client";import { cache } from "./cache";const client = new ApolloClient({cache,uri: "http://localhost:4000/graphql",});
The ApolloClient
constructor requires two parameters:
- The
uri
of our GraphQL server (in this caselocalhost:4000/graphql
). - An instance of
InMemoryCache
to use as the client'scache
. We import this instance from thecache.ts
file.
In just these few lines of code, our client is ready to fetch data!
Make your first query
Before we integrate Apollo Client with a React view layer, let's try sending a query with vanilla JavaScript.
- Add the following to the bottom of
index.tsx
:
// ...ApolloClient instantiated here...client.query({query: gql`query TestQuery {launch(id: 56) {idmission {name}}}`,}).then((result) => console.log(result));
Make sure your GraphQL server is running. If you didn't complete the server portion of the tutorial, you can run the finished server by running the following commands from
final/server
:npm installnpm startFrom
start/client
, runnpm start
to build and run your client app. When the build finishes, your browser opens tohttp://localhost:3000/
automatically.When the index page opens, you won't see any content just yet. Open up the console in your browser's developer tools. You'll see a logged
Object
that contains your server's response to your query. The data you requested is contained in the object'sdata
field, and the other fields provide metadata about the state of the request.
You can also open the Network tab in your browser's developer tools and refresh the page to view the shape of the request that Apollo Client makes to execute your query (it's a POST request to localhost:4000
).
- Go ahead and delete the
client.query()
call you just added, along with the unnecessarygql
import.
Now, let's go integrate our client with React!