Overview
We now have schema checks in our toolbelt, so let's see them put into action for the changes in the listings
subgraph.
In this lesson, we will:
- Review the code changes in the local dev environment
- Review value types and the
@shareable
directive in a schema - Learn how to run a schema check with Rover locally
Reviewing the mockup
Let's take a look at what we need for Project Galactic Coordinates again.
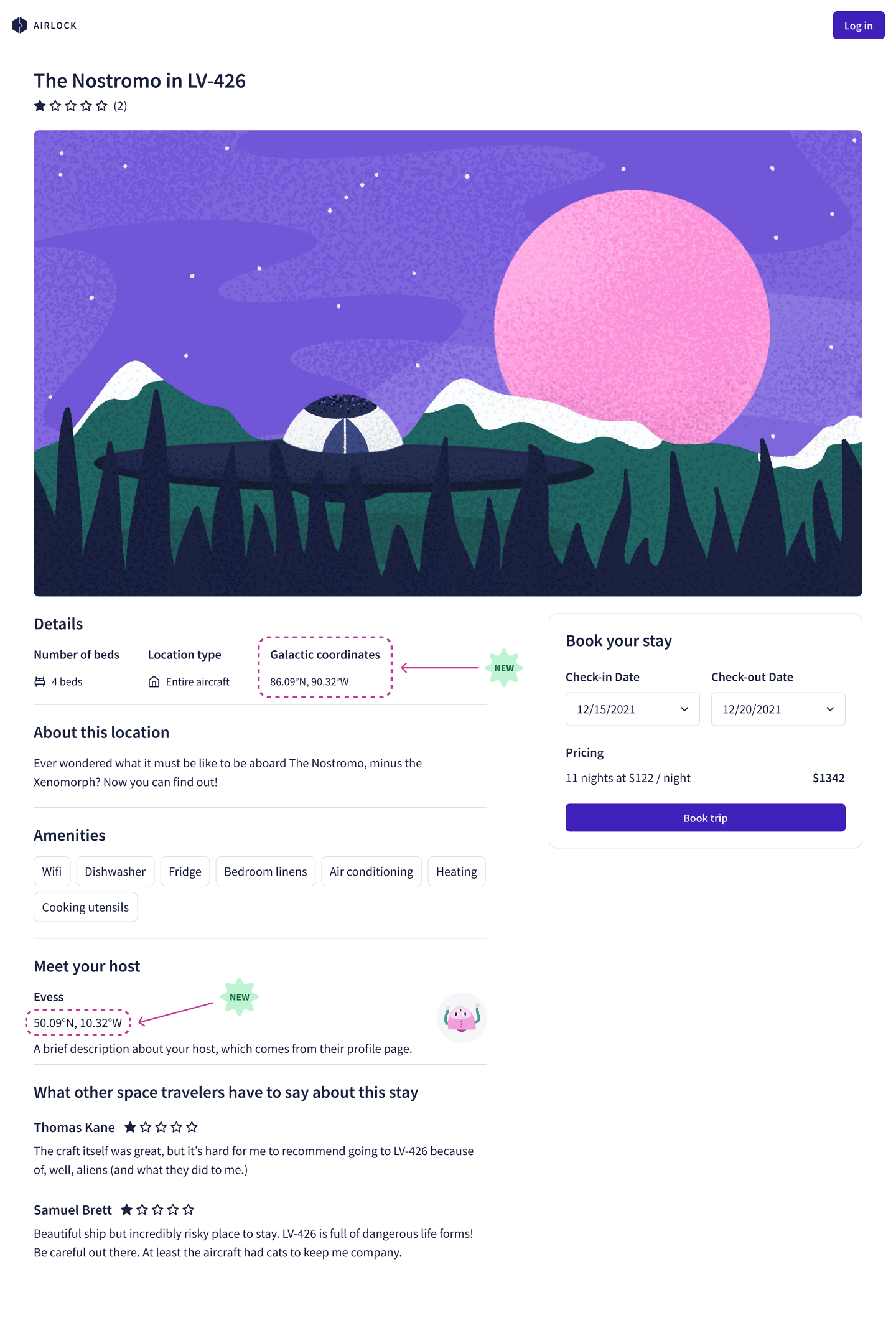
We need the listing details page to display the galactic coordinates of a listing and its host. The galactic coordinates are shown as a combination of latitude and longitude values.
Note: You won't be coding along with the course, but pay attention to the steps and concepts we're covering as we implement these code changes!
The Airlock team has two people working on this feature, one from each subgraph team:
- 👩🏽🏫 Lisa from the Listings team
- 👩🏽🚀 Achilles from the Accounts team
We'll be helping them incorporate what we've learned in the previous lessons (schema checks and graph variants) into their development process!
Changes in the local
development environment
👩🏽🏫 Lisa from the Listings team has already started to work on this feature by making additions to the schema, resolvers, and data sources. Let's take a look at what they've worked on to get this feature off the ground.
The listings
subgraph has a new type: GalacticCoordinates
. This object type has a latitude and longitude (both non-nullable Float
s).
"Coordinates in the galaxy"type GalacticCoordinates {latitude: Float!longitude: Float!}
The GalacticCoordinates
type is an example of a value type. Value types are types that are reused and shared across multiple subgraphs. These types can be object types, enums, unions, or interfaces. We've seen an example of an interface as a value type in Voyage II.
Right now, the GalacticCoordinates
object type is only used in the listings
subgraph, but remember that we'll also want to show the host's location, which belongs in the accounts
subgraph. The @shareable
directive will enable both subgraphs to resolve this type.
Note: If you completed Voyage II, we briefly covered the @shareable
directive in our discussion on field sharing. Note that value types that are object types (such as GalacticCoordinates
) need to use the @shareable
directive, but interfaces (such as what we covered in Voyage II) do not.
Lisa will need to add the @shareable
directive to the GalacticCoordinates
type.
"Coordinates in the galaxy"type GalacticCoordinates @shareable {latitude: Float!longitude: Float!}
Finally, Lisa also added one more field to the Listing
entity: coordinates
. This field returns the GalacticCoordinates
type.
type Listing @key(fields: "id") {# … other listing fields"Where this listing is located in the galaxy"coordinates: GalacticCoordinates}
Lisa has also implemented the code for both the resolvers and data sources to retrieve the correct data for this new field.
Awesome, these changes should get us started! Let's revisit the CI workflow for the next step: pushing the code to GitHub and opening up a PR.
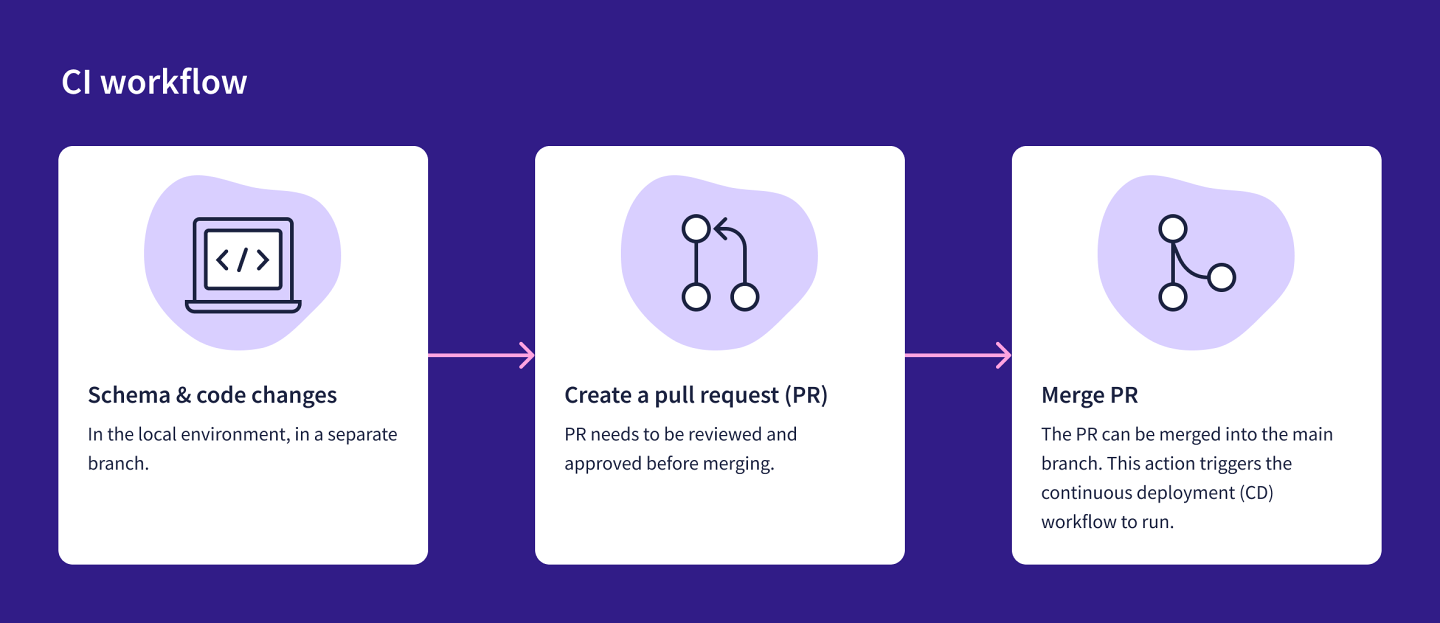
But wait! Don't forget we have a new item in our toolbelt: schema checks. We can run a schema check locally before pushing up our code. This will enable us to catch and address any errors related to composition or existing operations.
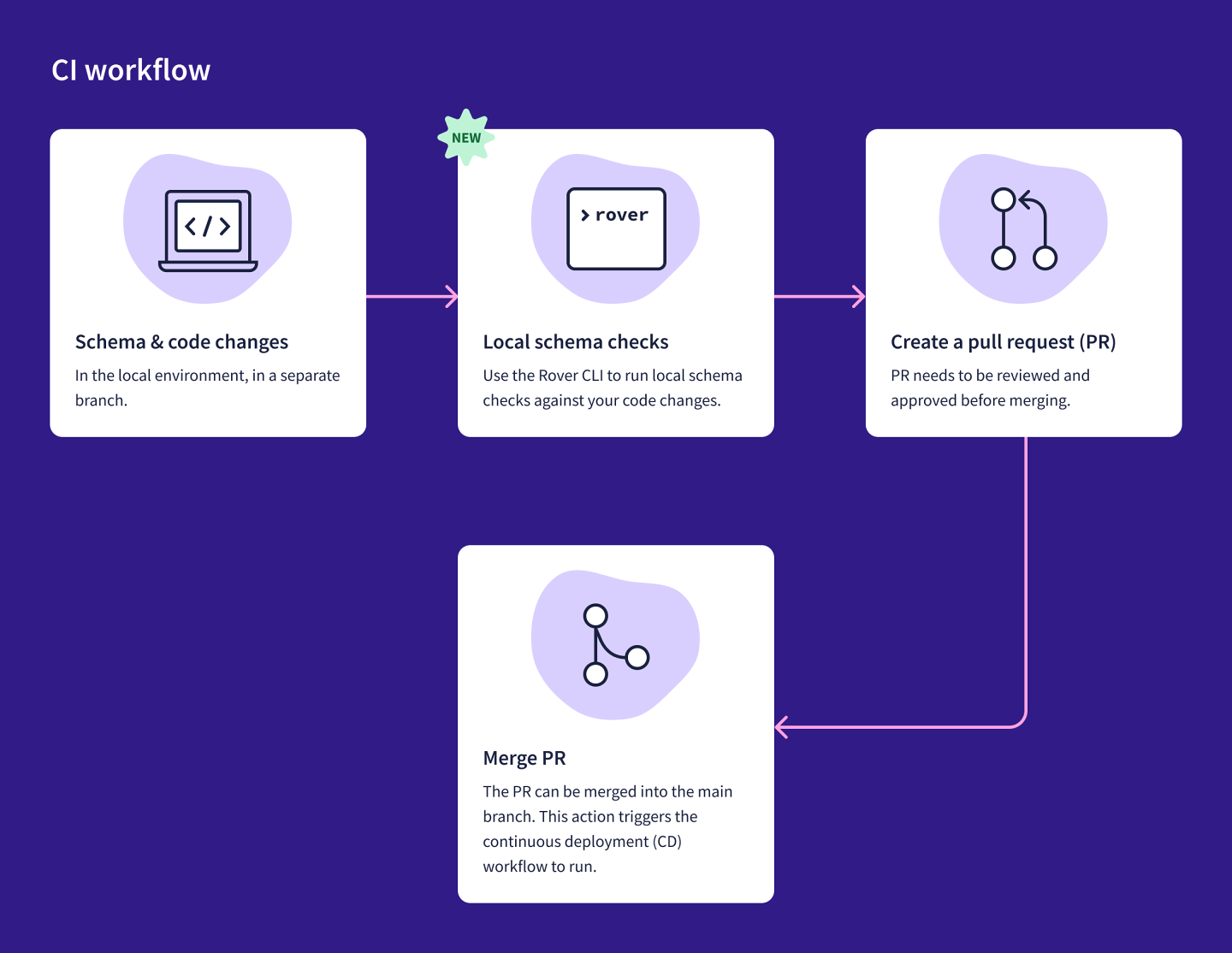
Running a local schema check
To run a local schema check, we'll use the rover subgraph check
command with the following parameters:
rover subgraph check <GRAPH_NAME>@<GRAPH_VARIANT> \--schema <SCHEMA_FILE_PATH> \--name <SUBGRAPH_NAME>
We'll want to check our local subgraph changes against the staging
variant, giving it the path to our listings.graphql
schema file and the name of the subgraph, listings
. After replacing the parameters with our subgraph's values, the command looks like this:
rover subgraph check airlock-managed-fed@staging \--schema "listings.graphql" \--name listings
Because Airlock is a supergraph, build checks will run first, then operation checks.
After the process completes, we can see a report of the schema changes. The terminal output shows the following:
Checking the proposed schema for subgraph listings against airlock-managed-fed@stagingCheck Result:Compared 4 schema changes against 16 operations┌────────┬─────────────┬─────────────────────────────────────────────────────┐│ Change │ Code │ Description │├────────┼─────────────┼─────────────────────────────────────────────────────┤│ PASS │ TYPE_ADDED │ type `GalacticCoordinates`: created │├────────┼─────────────┼─────────────────────────────────────────────────────┤│ PASS │ FIELD_ADDED │ type `GalacticCoordinates`: field `latitude` added │├────────┼─────────────┼─────────────────────────────────────────────────────┤│ PASS │ FIELD_ADDED │ type `GalacticCoordinates`: field `longitude` added │├────────┼─────────────┼─────────────────────────────────────────────────────┤│ PASS │ FIELD_ADDED │ type `Listing`: field `coordinates` added │└────────┴─────────────┴─────────────────────────────────────────────────────┘
The first column indicates whether each change passed or failed the check. The second column indicates the type of change we made, such as TYPE_ADDED
or FIELD_ADDED
. The last column provides a more detailed description of the change, such as what exact type was created and what field was added under the type.
Awesome, we have no errors! We can tell that the build check passed, because each row in the output table has a PASS
status. The schema changes were also compared against existing client operations, and no breaking changes were detected.
Let's think back to our development process. We've done the first step, and we've even improved our flow by adding in a local schema check.
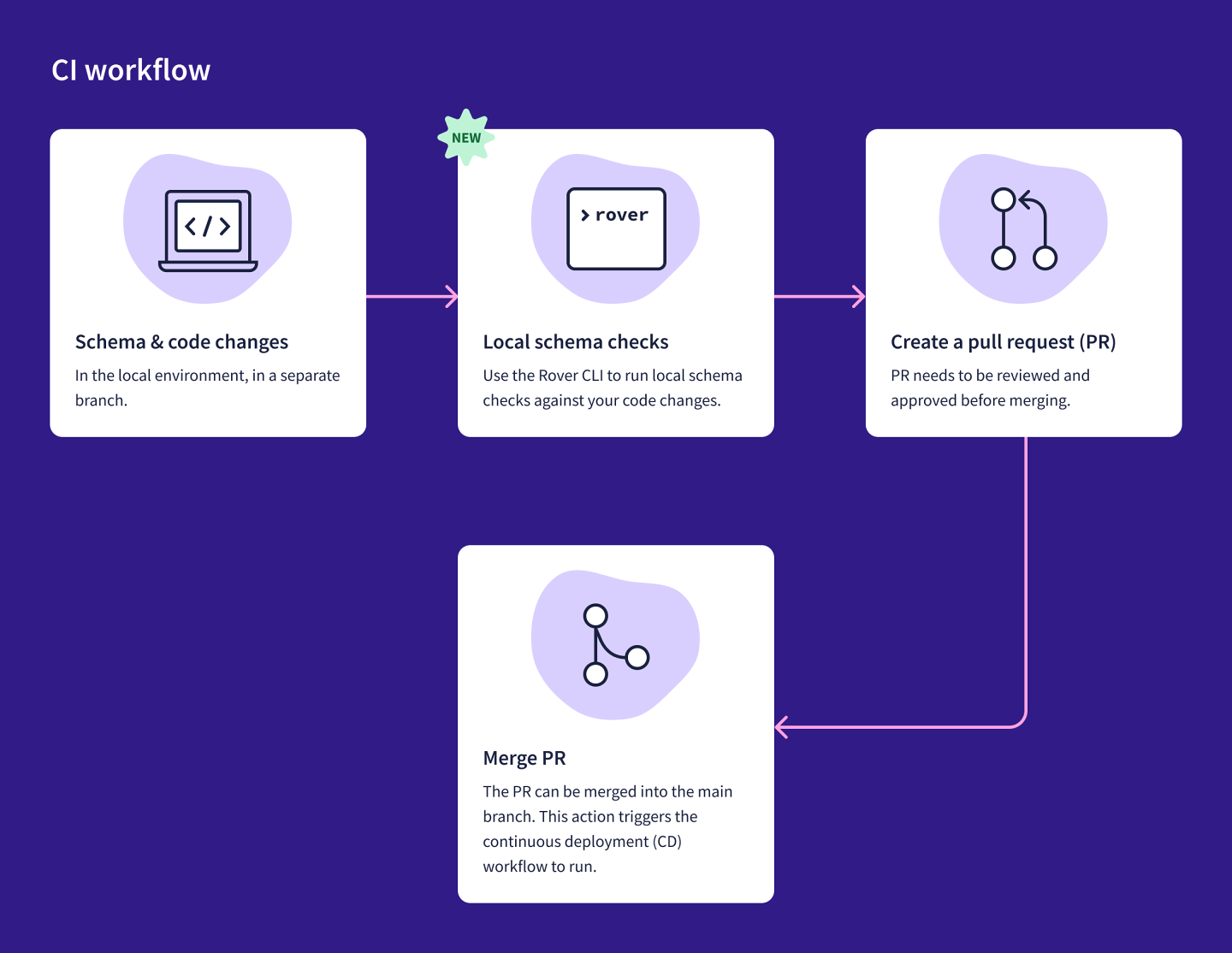
Practice
rover subgraph check
command need?rover subgraph check
command run on a non-federated graph?Key takeaways
- We use the
rover subgraph check
command to perform schema checks locally. - The
@shareable
directive enables multiple subgraphs to resolve a particular object field (or set of object fields).
Up next
Let's continue to improve our workflow. In the next lesson, we'll add schema checks to our CI pipeline so that they can run automatically whenever a PR is created.
Share your questions and comments about this lesson
Your feedback helps us improve! If you're stuck or confused, let us know and we'll help you out. All comments are public and must follow the Apollo Code of Conduct. Note that comments that have been resolved or addressed may be removed.
You'll need a GitHub account to post below. Don't have one? Post in our Odyssey forum instead.